Asynchronous Programming in Node.js
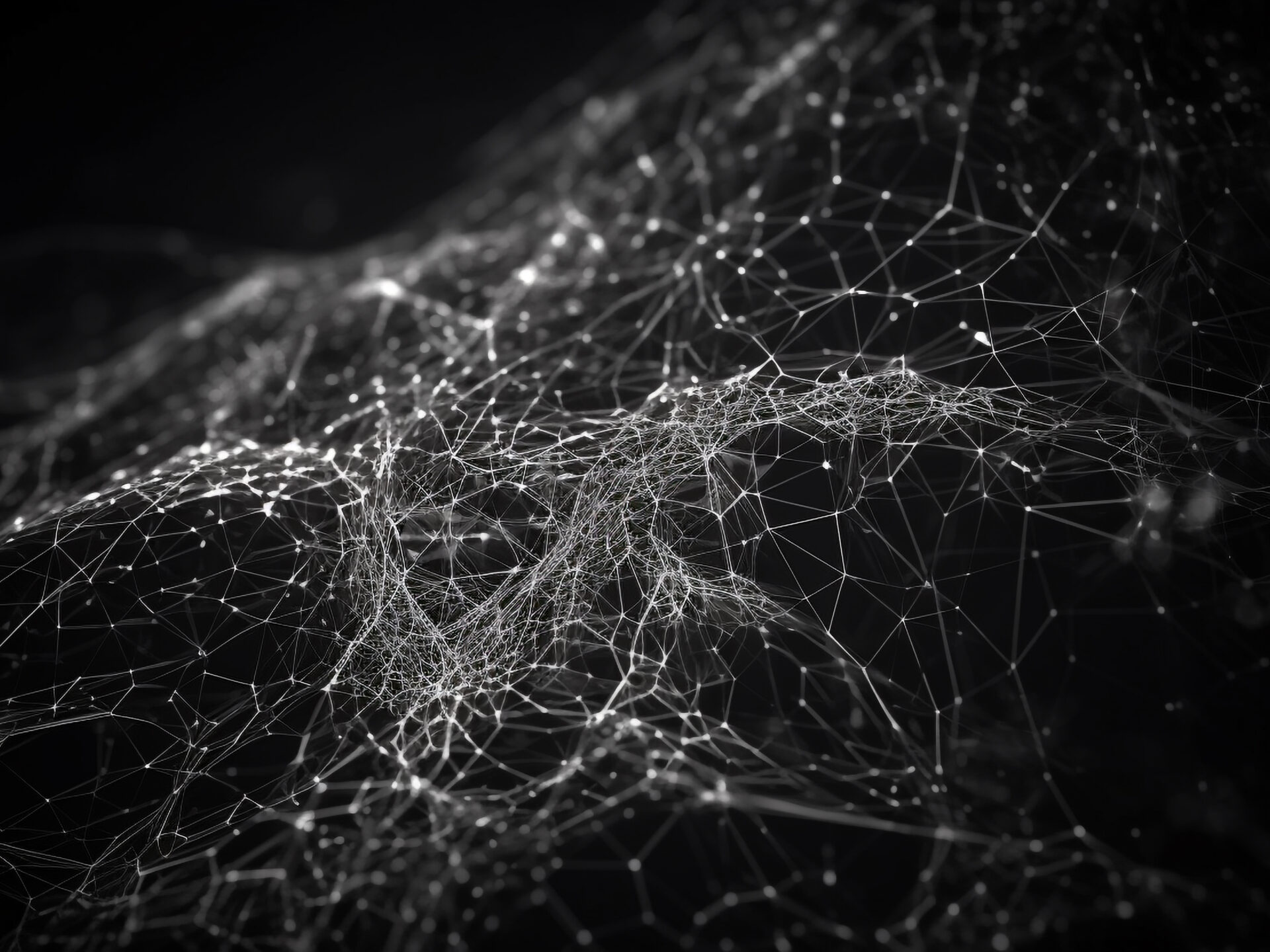
Asynchronous programming is one of the most important concepts in Node.js due to its non-blocking nature. This makes Node.js highly efficient for handling multiple operations at once without waiting for previous tasks to complete. Understanding how Node.js handles asynchronous operations using callbacks, promises, and the more modern async/await is crucial for building performant applications.
Callbacks: The Foundation of Async in Node.js
When Node.js was first introduced, callbacks were the primary way to handle asynchronous code. A callback is a function passed as an argument to another function, which is then executed once the asynchronous operation is complete. Here’s an example of a basic callback structure:
const fs = require('fs');
fs.readFile('file.txt', 'utf8', function(err, data) {
if (err) {
return console.error(err);
}
console.log(data);
});
In the example above, fs.readFile
performs an asynchronous read operation, and the callback function is called when the file read operation is completed, either with an error (err
) or the file’s contents (data
).
While callbacks are effective, they can lead to a problem known as callback hell, where callbacks are nested within other callbacks, leading to code that is difficult to read and maintain:
fs.readFile('file1.txt', 'utf8', function(err, data1) {
fs.readFile('file2.txt', 'utf8', function(err, data2) {
fs.readFile('file3.txt', 'utf8', function(err, data3) {
// Continue processing...
});
});
});
Promises: A Cleaner Way
To avoid callback hell, promises were introduced in modern JavaScript as a more structured way to handle asynchronous operations. A promise represents a value that will be available in the future. It can either be fulfilled (resolved) or rejected. Here’s how you can handle asynchronous operations using promises:
const fs = require('fs').promises;
fs.readFile('file.txt', 'utf8')
.then(data => {
console.log(data);
})
.catch(err => {
console.error(err);
});
In this example, .then()
is used to handle the resolved value of the promise, while .catch()
is used to catch any errors. Promises allow chaining and avoid deeply nested structures, making code much more readable.
Additionally, multiple promises can be run concurrently using Promise.all()
, which will resolve only when all promises are fulfilled:
Promise.all([
fs.readFile('file1.txt', 'utf8'),
fs.readFile('file2.txt', 'utf8'),
fs.readFile('file3.txt', 'utf8')
])
.then(results => {
console.log(results);
})
.catch(err => {
console.error(err);
});
async/await: Simplifying Asynchronous Code
While promises significantly improved asynchronous code, async/await provides an even more straightforward syntax by allowing you to write asynchronous code that looks synchronous. async
functions return promises, and await
pauses the execution until the promise is resolved. Here’s how to refactor the promise example using async/await:
async function readFileAsync() {
try {
const data = await fs.readFile('file.txt', 'utf8');
console.log(data);
} catch (err) {
console.error(err);
}
}
readFileAsync();
With async/await, the code becomes linear, making it easier to read and maintain. It also eliminates the need for .then()
and .catch()
chains, allowing you to use try...catch
for error handling instead.
Combining Multiple async/await Operations
Similar to Promise.all()
, you can combine multiple asynchronous operations with async/await. Here’s an example:
async function readMultipleFiles() {
try {
const [data1, data2, data3] = await Promise.all([
fs.readFile('file1.txt', 'utf8'),
fs.readFile('file2.txt', 'utf8'),
fs.readFile('file3.txt', 'utf8')
]);
console.log(data1, data2, data3);
} catch (err) {
console.error(err);
}
}
readMultipleFiles();
In this example, Promise.all()
is used with await
to run the file reading operations concurrently, further improving performance by running them in parallel rather than sequentially.
Asynchronous programming is at the heart of Node.js, making it powerful for I/O-heavy operations. Starting with callbacks, evolving through promises, and finally to async/await, JavaScript now provides multiple ways to handle asynchronous code effectively. Understanding these concepts is key to writing cleaner, more efficient, and maintainable Node.js applications.