How React Determines Re-rendering Of A Component
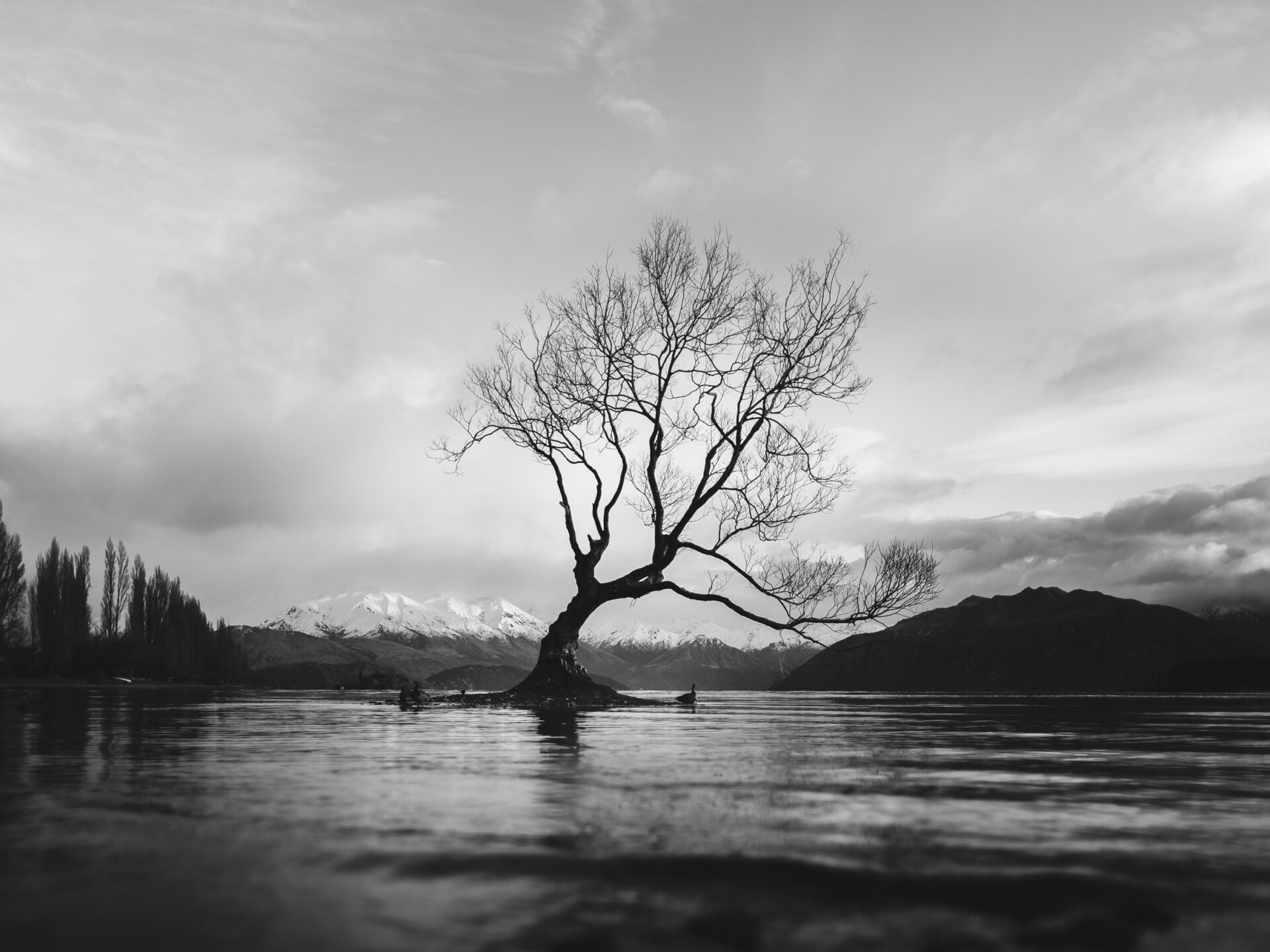
React is a library for building UI interfaces best known for its fast rendering performance. Its speed is made possible by its use of a virtual DOM (Document Object Model), a virtual representation of the actual DOM. But understanding how React determines when to re-render a component is essential for optimizing performance and building efficient applications.
React re-renders components in response to changes in state or props. When a component’s state changes, React marks it as needing an update. The same happens when a parent component passes new props to a child component. But not every change triggers a full re-render; React optimizes the process by selectively re-rendering only the necessary parts of the UI.
The Virtual DOM and Reconciliation
The key to React’s performance lies in its virtual DOM. When a component’s state or props change, React doesn’t immediately update the actual DOM. Instead, it first updates the virtual DOM, which is a lightweight copy of the actual DOM. React then uses a process called reconciliation to compare the virtual DOM with the previous version. This comparison allows React to identify exactly which parts of the DOM need to be updated, avoiding unnecessary changes and keeping the UI responsive.
How React Decides to Re-render
React determines whether to re-render a component by using the following steps:
- State or Props Change: When a component’s state or props change, React marks the component for re-rendering.
- ShouldComponentUpdate: React calls the
shouldComponentUpdate
lifecycle method (or its hook equivalent,React.memo
) to decide if re-rendering is needed. By default, React will re-render whenever state or props change, but you can override this behavior to prevent unnecessary renders. - Reconciliation: React performs a “diff” between the old and new virtual DOM trees to determine which parts of the DOM have changed. This process is efficient, as React only updates the DOM nodes that differ from the previous render.
- DOM Updates: After reconciliation, React updates the real DOM, applying only the minimal set of changes required to reflect the updated component.
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
shouldComponentUpdate(nextProps, nextState) {
// Only re-render if count is different
return nextState.count !== this.state.count;
}
render() {
return <div>{this.state.count}</div>;
}
}
In this example, the shouldComponentUpdate
method is used to control whether the component should re-render. By comparing the current state with the next state, React can skip re-rendering if the count
value hasn’t changed, improving performance.
Optimizing Re-renders with React.memo
For functional components, React provides React.memo, a higher-order component that allows you to prevent unnecessary re-renders. It works similarly to shouldComponentUpdate
, but for functional components:
const MyComponent = React.memo(({ count }) => {
return <div>{count}</div>;
});
Now, MyComponent
will only re-render if the count
prop changes, helping optimize performance in large applications.
Conclusion
React’s re-rendering behavior is driven by changes in state and props, but thanks to the virtual DOM and reconciliation process, only the necessary parts of the UI are updated. By leveraging lifecycle methods like shouldComponentUpdate
and tools like React.memo
, developers can further optimize re-rendering and ensure their applications run efficiently.