Building a REST API with Go
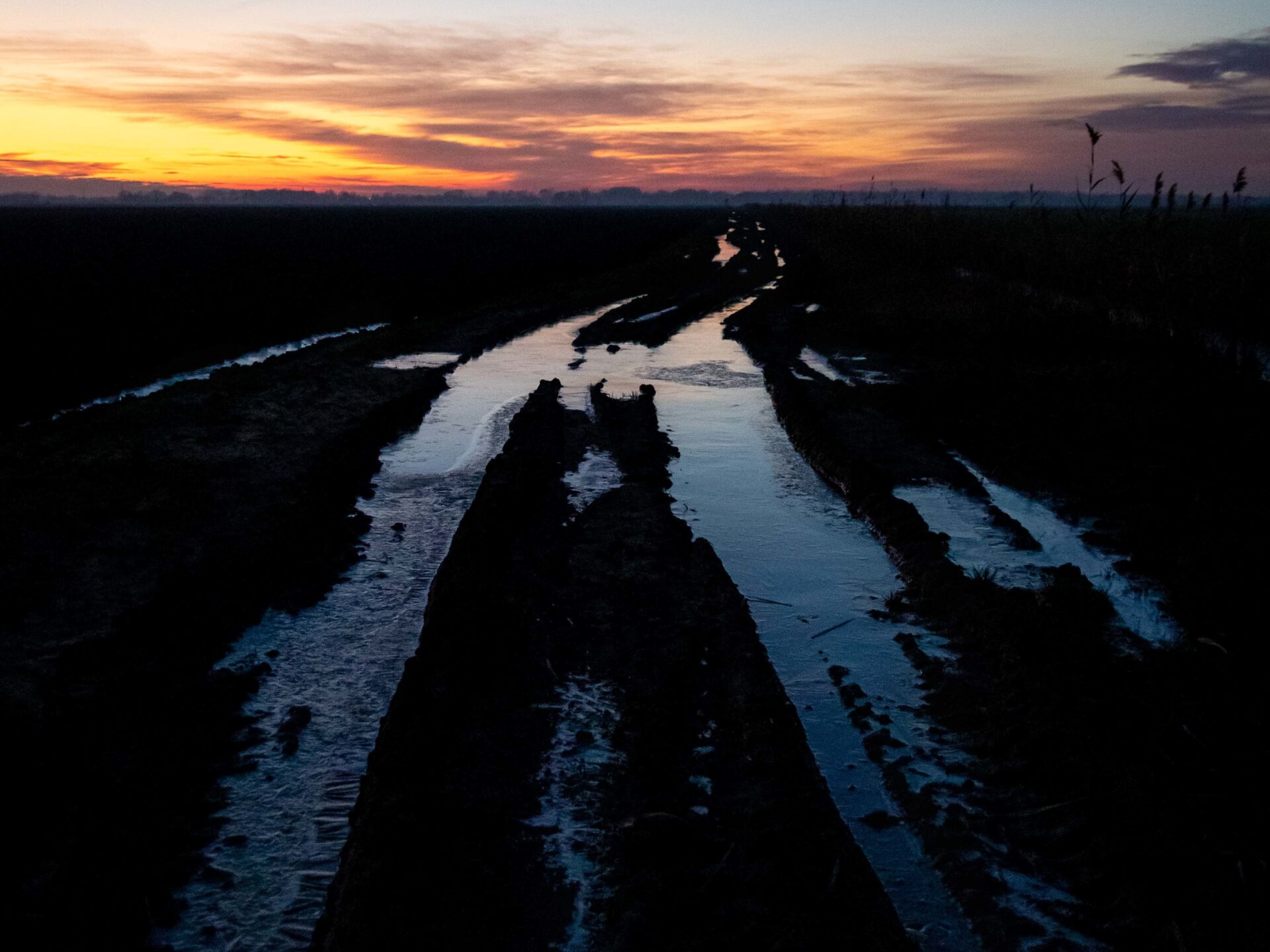
Creating a REST API can be a rewarding experience that enhances your skills and enables your applications to interact with other services. Go, known for its simplicity and efficiency, is a fantastic choice for building RESTful APIs. This guide will take you through the essentials of creating a REST API using Go.
Why Go for REST APIs?
Go is designed for high performance and scalability, making it ideal for developing REST APIs. Its built-in concurrency features, such as goroutines, allow handling multiple requests simultaneously without a hitch. Plus, its strong standard library and third-party packages simplify the development process.
Setting Up Your Go Environment
First, ensure you have Go installed on your machine. You can download it from the official Go website. Once installed, you can check your installation by running:
go version
Next, create a new directory for your project:
mkdir go-rest-api
cd go-rest-api
go mod init go-rest-api
Creating the API Structure
Let’s create a simple API to manage a list of users. Start by creating a main.go file:
package main
import (
"encoding/json"
"net/http"
)
type User struct {
ID int `json:"id"`
Name string `json:"name"`
}
var users []User
func getUsers(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(users)
}
func main() {
users = append(users, User{ID: 1, Name: "John Doe"})
http.HandleFunc("/users", getUsers)
http.ListenAndServe(":8080", nil)
}
Running API
To run your API, execute the following command:
go run main.go
You can test your API by visiting http://localhost:8080/users
in your browser or using a tool like Postman.
Adding More Functionality
To make your API more functional, consider adding endpoints for creating, updating, and deleting users. This can be achieved using the HTTP methods POST, PUT, and DELETE.