Unit Testing in Go
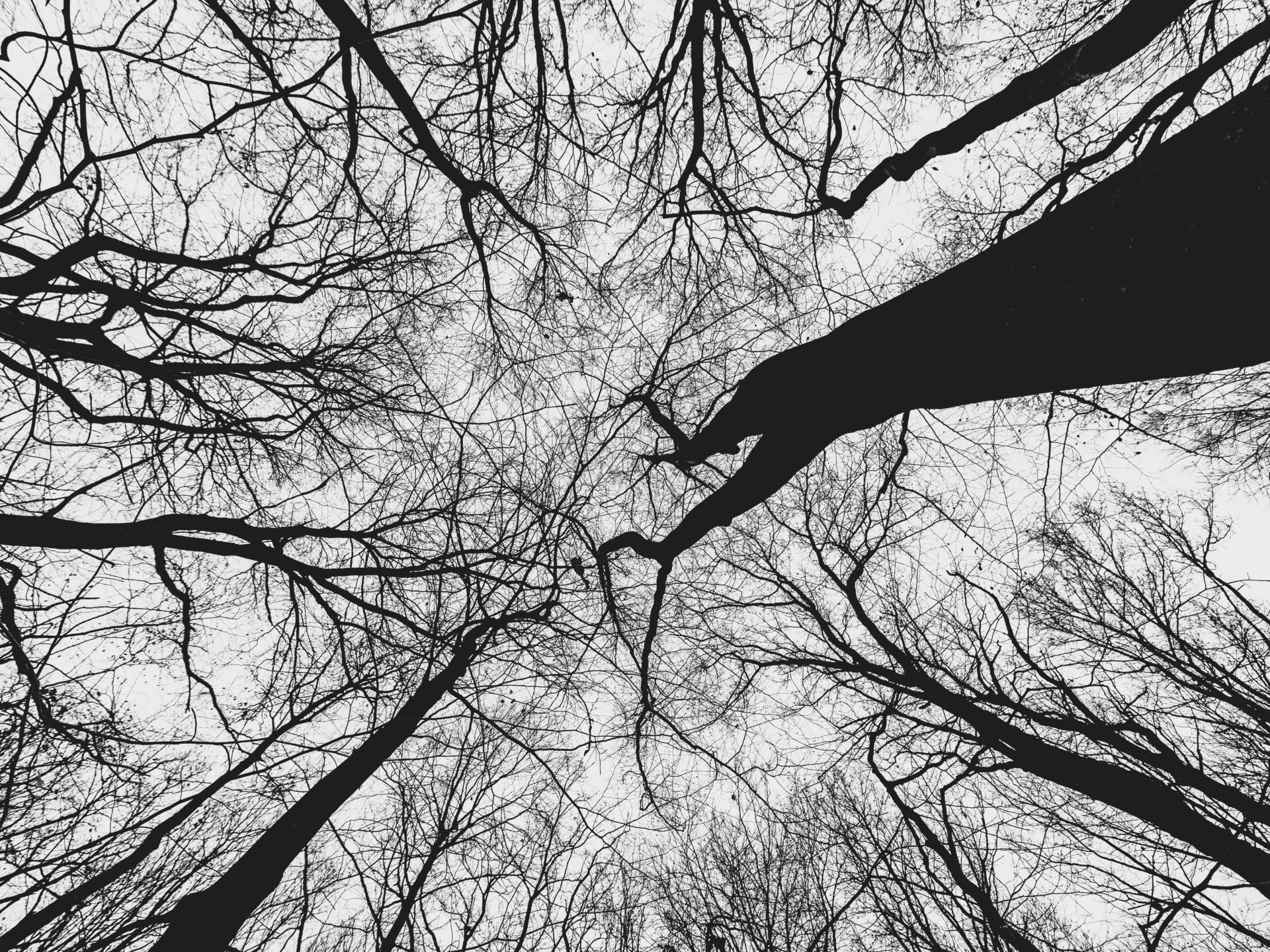
Unit tests are essential for enhancing the overall quality of your codebase by allowing you to verify each unit of your application independently. A unit can be a function, method, or component that is isolated from the rest of your code. Writing unit tests encourages you to create testable code and modularize your codebase into smaller, more manageable pieces.
Go provides a robust testing framework built right into the language, making it straightforward to write unit tests. The standard testing
package allows you to create tests with minimal configuration, unlike some other languages that require extensive setup.
Why Go’s Testing Framework Stands Out
In my experience with various testing frameworks in other languages, I often encountered the need for significant boilerplate code and complex configuration just to get started. Many frameworks require you to pick additional assertion libraries and test runners, which can be cumbersome and time-consuming.
Go’s approach simplifies this process. With Go, you write your test functions in the same package as the code you’re testing, and the go test
command handles everything for you. This direct approach keeps your test suite clean and easy to manage, allowing you to focus on writing tests rather than configuring your environment.
The Structure of Tests in Go
In Go, tests are written in files ending with _test.go
. Each test function starts with Test
, followed by a descriptive name, and takes a pointer to testing.T
as its argument. Here’s a simple example:
package main
import (
"testing"
)
func Add(a, b int) int {
return a + b
}
func TestAdd(t *testing.T) {
result := Add(2, 3)
expected := 5
if result != expected {
t.Errorf("Expected %d, but got %d", expected, result)
}
}
In this example, the Add
function is tested within the TestAdd
function. If the result does not match the expected output, an error is logged using t.Errorf
.
Table-Driven Tests
Go promotes a style of testing known as table-driven tests, which allows you to run the same test logic with different input values. Here’s how you can implement this:
func TestAddVarious(t *testing.T) {
tests := []struct {
a, b int
expected int
}{
{2, 3, 5},
{0, 0, 0},
{-1, 1, 0},
{100, 200, 300},
}
for _, test := range tests {
result := Add(test.a, test.b)
if result != test.expected {
t.Errorf("Add(%d, %d) = %d; expected %d", test.a, test.b, result, test.expected)
}
}
}
By organizing your tests this way, you can easily add more test cases without duplicating code.
Benchmark Testing
Go also makes it simple to run benchmark tests using functions prefixed with Benchmark
. Here’s an example:
func BenchmarkAdd(b *testing.B) {
for i := 0; i < b.N; i++ {
Add(1, 2)
}
}
To run your benchmarks, you can execute the following command:
go test -bench=.
This will give you insight into the performance of your code.
Running Your Tests
Executing your tests is straightforward. In your project’s root directory, simply run:
go test
This command will automatically discover and execute all the tests in your package, providing you with a clear report of their status.
Conclusion
As of now, Go’s testing framework offers a simple yet powerful way to ensure the reliability of your applications. By making it easy to write and run tests, Go allows developers to focus more on building features rather than getting bogged down in the testing setup. The ability to run tests from the command line with minimal configuration is a significant advantage, enabling you to maintain a clean and effective testing workflow.
Go’s testing capabilities help you write better unit tests that catch bugs early and improve the overall design of your code. With its straightforward approach, you can seamlessly integrate testing into your development process, ultimately leading to more robust applications.