File System in Node.js
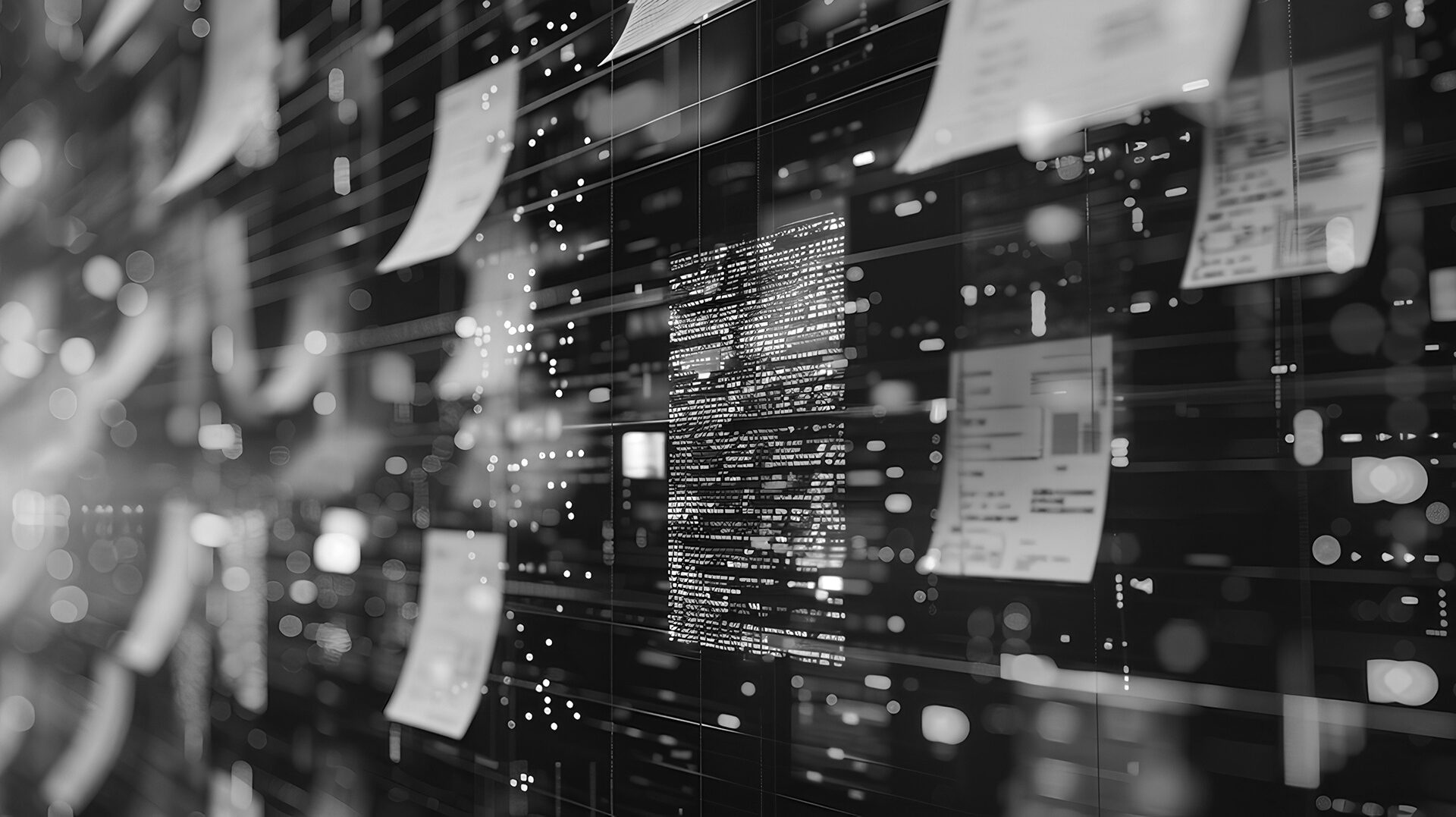
Node.js provides a powerful API for interacting with the file system, enabling tasks such as reading, writing, appending, and deleting files directly through the built-in fs
module. This is critical for building efficient backend applications or scripts that need to manipulate files. Let’s dive deeper into how to leverage this functionality.
Getting Started
First things first, to use the file system, you need to require the fs
module:
const fs = require('fs');
This single line grants access to a wide range of file handling capabilities.
Reading Files
To read the content of a file, the fs.readFile
method is your go-to. Here’s a practical example:
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
In this case, example.txt
is read asynchronously. The utf8
argument ensures proper character encoding, and the callback handles both success (logging the content) and failure (throwing an error). It’s important to manage such errors to prevent issues like trying to read non-existent files from crashing your application.
Writing Files
To write data into a file, use fs.writeFile
. The following snippet demonstrates how to create and populate a file:
fs.writeFile('newFile.txt', 'Hello, Node!', 'utf8', (err) => {
if (err) throw err;
console.log('File written successfully!');
});
This will create newFile.txt
and write “Hello, Node!” to it. If the file exists, its content will be replaced entirely, so be mindful of potential data loss.
Appending Data
When you want to add data without overwriting the file, fs.appendFile
is the correct approach:
fs.appendFile('log.txt', 'New log entry\n', 'utf8', (err) => {
if (err) throw err;
console.log('Content added!');
});
This operation ensures that new content (“New log entry”) is appended to log.txt
while preserving its previous data.
Deleting Files
To remove a file from the system, Node.js offers fs.unlink
:
fs.unlink('oldFile.txt', (err) => {
if (err) throw err;
console.log('File deleted!');
});
This deletes oldFile.txt
permanently. Handling errors is crucial here, particularly if you’re dealing with non-existent or inaccessible files.
Working with Directories
Node.js also supports directory manipulation. To create a directory:
fs.mkdir('newFolder', (err) => {
if (err) throw err;
console.log('Folder created!');
});
To read the contents of a directory:
fs.readdir('.', (err, files) => {
if (err) throw err;
console.log(files);
});
This reads the current directory (.
) and returns a list of files and subdirectories.