Understanding the Node.js Event Loop
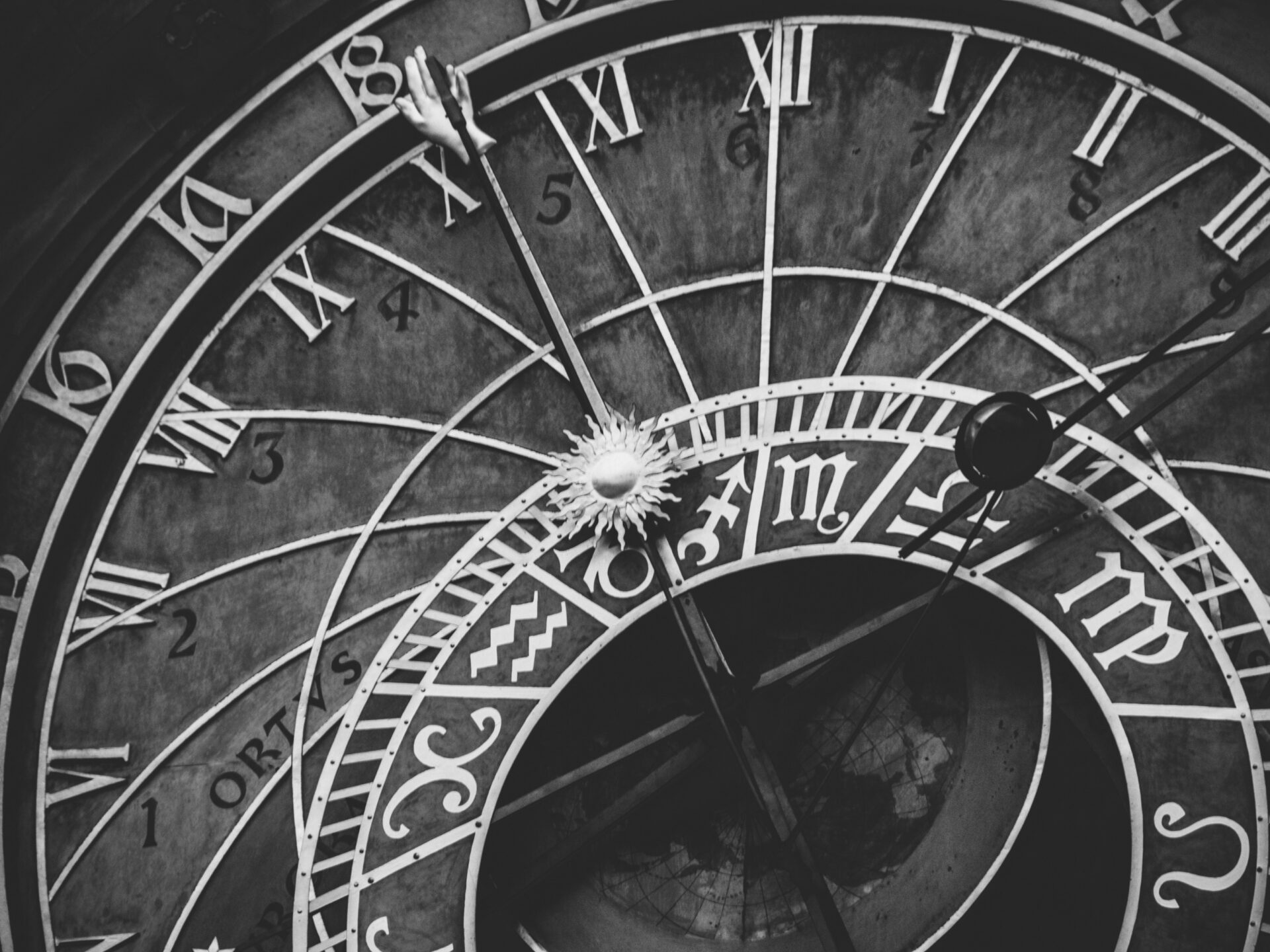
If you’re building Node.js applications, one of the most important concepts to understand is Node.js’ concurrency model for handling I/O operations and its use of callback functions. Node.js is single-threaded but achieves asynchronous behavior through the event loop, allowing it to handle multiple I/O operations concurrently without blocking the main execution thread.
The event loop is essentially a mechanism that continuously checks for tasks, such as file operations or network requests, and delegates them for execution while freeing up the main thread for other operations. Once these tasks are complete, their associated callback functions are placed into the queue for execution. This architecture is key to how Node.js handles high throughput with non-blocking I/O.
To better understand this, let’s break down how the event loop works:
- Timers Phase: Executes callbacks scheduled by
setTimeout()
andsetInterval()
. - Pending Callbacks Phase: Executes I/O callbacks that were deferred to the next loop iteration.
- Idle/Prepare Phase: Internal operations that are rarely of concern to developers.
- Poll Phase: Retrieves new I/O events, executing related callbacks.
- Check Phase: Executes callbacks from
setImmediate()
. - Close Callbacks Phase: Executes
close
event callbacks, like when a socket is closed.
Example
setTimeout(() => {
console.log('Timeout callback');
}, 0);
setImmediate(() => {
console.log('Immediate callback');
});
console.log('Main execution');
In the above code, even though setTimeout
has a delay of 0 milliseconds, the setImmediate
callback may still run first due to the different phases of the event loop. The output might be:
Main execution
Immediate callback
Timeout callback
The event loop makes Node.js highly efficient in handling asynchronous operations. By leveraging this, you can build scalable, high-performance applications that handle a large number of simultaneous connections.